Build Docker Container for Node.js and MySQL Based Application
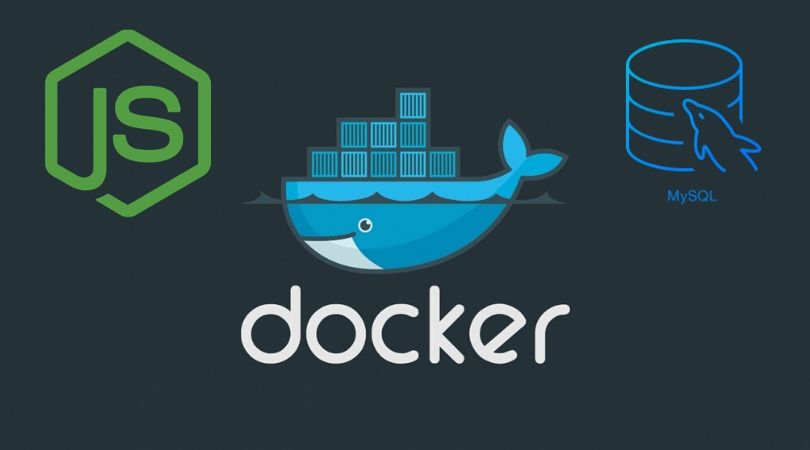
Lets talk very simple. You are a Node.js developer and building your application with MySQL database. You want to containerize your application using Docker. Yes, this blog will help you to find out how.
For PHP and MySQL, you may read our blog on Build Docker Container for PHP 7 and MySQL Based Application
Watch YouTube video tutorial guide on how to build Docker containers for Node.js and MySQL database.
Watch the above video to know the details of the concept before you read further.
Step 1: Write the Node.js code
The index.js
file will look like as below:
var mysql = require('mysql');
var express = require('express');
var app = express();
var port = process.env.PORT || 8005;
var responseStr = "MySQL Data:";
app.get('/',function(req,res){
var mysqlHost = process.env.MYSQL_HOST || 'localhost';
var mysqlPort = process.env.MYSQL_PORT || '3306';
var mysqlUser = process.env.MYSQL_USER || 'root';
var mysqlPass = process.env.MYSQL_PASS || 'root';
var mysqlDB = process.env.MYSQL_DB || 'node_db';
var connectionOptions = {
host: mysqlHost,
port: mysqlPort,
user: mysqlUser,
password: mysqlPass,
database: mysqlDB
};
console.log('MySQL Connection config:');
console.log(connectionOptions);
var connection = mysql.createConnection(connectionOptions);
var queryStr = SELECT * FROM MOE_ITEM_T;
connection.connect();
connection.query(queryStr, function (error, results, fields) {
if (error) throw error;
responseStr = '';
results.forEach(function(data){
responseStr += data.ITEM_NAME + ' : ';
console.log(data);
});
if(responseStr.length == 0)
responseStr = 'No records found';
console.log(responseStr);
res.status(200).send(responseStr);
});
connection.end();
});
app.listen(port, function(){
console.log('Sample mySQL app listening on port ' + port);
});
Here with this very simple example we are connecting to the MySQL database. We are using environment variables to pass the details here. You may choose different approach as suited for you.
var mysqlHost = process.env.MYSQL_HOST || 'localhost';
var mysqlPort = process.env.MYSQL_PORT || '3306';
var mysqlUser = process.env.MYSQL_USER || 'root';
var mysqlPass = process.env.MYSQL_PASS || 'root';
var mysqlDB = process.env.MYSQL_DB || 'node_db';
Rest of the code is self explanatory. It connects to the database and execute an SELECT
SQL query and finally returns the values of the ITEM_NAME
field from table MOE_ITEM_T
.
Step 2: Build the Docker file
Now create the docker file like below:
FROM node:8
WORKDIR /usr/src/app
COPY package*.json ./
RUN npm install
COPY . .
EXPOSE 8005
CMD [ "npm", "start" ]
Also the package.json file as below:
{
"name": "mysql-db-example",
"version": "1.0.0",
"description": "",
"main": "index.js",
"scripts": {
"start": "node index.js"
},
"author": "",
"license": "ISC",
"dependencies": {
"express": "^4.16.4",
"mysql": "^2.17.1"
}
}
Now build the docker image form the Docker
file with the below command:
sudo docker build . -t amakundu/nodejs-mysql-app
Change the amakundu
part as of your Docker Hub name.
Step 3: Build the Docker Compose File.
Create a file named docker-compose.yml
as below:
version: "3.2"
services:
nodejs:
build:
context: .
image: amakundu/nodejs-mysql-app
networks:
- frontend
- backend
environment:
- MYSQL_HOST=moe-mysql-app
- MYSQL_USER=moeuser
- MYSQL_PASS=moepass
- MYSQL_DB=moe_db
volumes:
- ./www/:/var/www/html/
ports:
- "30001:8005"
container_name: moe-nodejs-app
mysql:
image: mysql:5.7
networks:
- backend
environment:
- MYSQL_ROOT_PASSWORD=root
- MYSQL_USER=moeuser
- MYSQL_PASSWORD=moepass
- MYSQL_DATABASE=moe_db
container_name: moe-mysql-app
phpmyadmin:
image: phpmyadmin/phpmyadmin:4.7
depends_on:
- mysql
networks:
- backend
ports:
- "30002:80"
environment:
- PMA_HOST=moe-mysql-app
- PMA_PORT= 3306
volumes:
- /sessions
container_name: moe-phpmyadmin-app
networks:
frontend:
backend:
In the node-js
service, we are creating the container for our Node.js application's docker image.
mysql
service is spinning up the container from Docker standard MySQL database image mysql:5.7
Next service is the phpmyadmin which will allow us to access the MySQL database using an UI (User Interface). You may notice that the MySQL database host details are passed using the below two environment variables. Where "moe-mysql-app
" is the name of the MySQL service container.
PMA_HOST=moe-mysql-app
PMA_PORT= 3306
Step 4: Docker Compose run
sudo docker-compose up --build
The above command will spin up three containers. One for Node.js application. 2nd one is of MySQL and the third one for PHPMyAdmin.
Now access the PHPMyAdmin application from browser through URL http://localhost:30002/
Use the username and password used in mysql
service to login. Create the database node_db
and run the below script to create the test table and test data.
-- phpMyAdmin SQL Dump
-- version 4.7.9
-- https://www.phpmyadmin.net/
--
-- Generation Time: Dec 04, 2018 at 11:50 AM
-- Server version: 5.7.24
-- PHP Version: 7.2.2
SET SQL_MODE = "NO_AUTO_VALUE_ON_ZERO";
SET AUTOCOMMIT = 0;
START TRANSACTION;
SET time_zone = "+00:00";
/*!40101 SET @OLD_CHARACTER_SET_CLIENT=@@CHARACTER_SET_CLIENT */;
/*!40101 SET @OLD_CHARACTER_SET_RESULTS=@@CHARACTER_SET_RESULTS */;
/*!40101 SET @OLD_COLLATION_CONNECTION=@@COLLATION_CONNECTION */;
/*!40101 SET NAMES utf8mb4 */;
--
-- Database: `moe_db`
--
-- --------------------------------------------------------
--
-- Table structure for table `MOE_ITEM_T`
--
CREATE TABLE `MOE_ITEM_T` (
`ITEM_NAME` varchar(50) NOT NULL,
`ITEM_DESC` varchar(100) NOT NULL,
`ITEM_ONHAND` int(5) NOT NULL
) ENGINE=InnoDB DEFAULT CHARSET=utf8;
--
-- Dumping data for table `MOE_ITEM_T`
--
INSERT INTO `MOE_ITEM_T` (`ITEM_NAME`, `ITEM_DESC`, `ITEM_ONHAND`) VALUES
('TEST-ITEM-1', 'TEST-ITEM-DESC-1', 10),
('TEST-ITEM-2', 'TEST-ITEM-DESC-2', 20);
COMMIT;
/*!40101 SET CHARACTER_SET_CLIENT=@OLD_CHARACTER_SET_CLIENT */;
/*!40101 SET CHARACTER_SET_RESULTS=@OLD_CHARACTER_SET_RESULTS */;
/*!40101 SET COLLATION_CONNECTION=@OLD_COLLATION_CONNECTION */;
Step 4: Access your Node.js application
Since the Node.js application is also running up from the above docker-compose up
command, access the application in browser using URL http://localhost:30001/
You see the output from the application on browser as :
TEST-ITEM-1 : TEST-ITEM-2 :
You can view the code files in GitHub Repository.
Comments